Memento
The memento pattern
The memento pattern is a behavioral pattern and is for storing the state of an object instance without exposing the internals of the object (encapsulation).A structure diagram of the pattern is below with the Memento class storing the internals of the Originator, with the Caretaker class only able to manipulate the Originator but being able to return to a past state of the Originator.
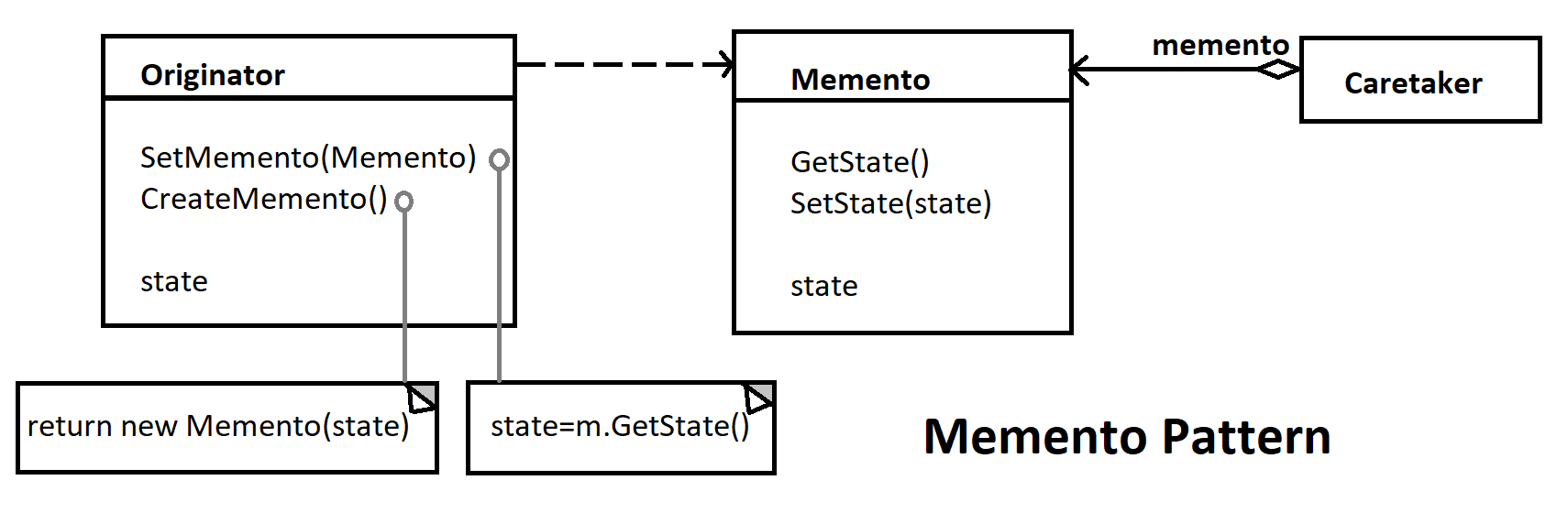
We'll start off with the Originator and I'm trying my hardest to avoid utilising a stack.
class Originator {
private List mementos = new List();
private int index = 0;
private State state;
public void SetState(State state) {
mementos.Add(new Memento(this.state));
index++;
this.state = state;
}
public State GetState() {
return state;
}
public void Undo() {
if (index>0) state = mementos[--index].GetState();
}
}
private List
private int index = 0;
private State state;
public void SetState(State state) {
mementos.Add(new Memento(this.state));
index++;
this.state = state;
}
public State GetState() {
return state;
}
public void Undo() {
if (index>0) state = mementos[--index].GetState();
}
}
Here's the Memento class which I've made immutable:
public class Memento
private State state;
public Memento(State state) {
this.state = state;
}
internal State GetState() {
return state;
}
}
private State state;
public Memento(State state) {
this.state = state;
}
internal State GetState() {
return state;
}
}
The State object has the following implementation, and (again) is immutable:
public class State {
public int Level { get; }
public string Message { get; }
public State(int level, string message) {
Level = level;
Message = message;
}
public override string ToString() {
return "Level is " + Level + " with message " + Message;
}
}
public int Level { get; }
public string Message { get; }
public State(int level, string message) {
Level = level;
Message = message;
}
public override string ToString() {
return "Level is " + Level + " with message " + Message;
}
}
The Caretaker is the console application in this instance and is:
class Caretaker {
static void Main(string[] args) {
Originator originator = new Originator();
originator.SetState(new State(1, "state 1"));
Console.WriteLine(originator.GetState());
originator.SetState(new State(2, "state 2"));
Console.WriteLine(originator.GetState());
originator.SetState(new State(3, "state 3"));
Console.WriteLine(originator.GetState());
originator.Undo();
originator.Undo();
Console.WriteLine(originator.GetState());
originator.SetState(new State(1, "new state 1"));
Console.WriteLine(originator.GetState());
Console.ReadKey();
}
}
static void Main(string[] args) {
Originator originator = new Originator();
originator.SetState(new State(1, "state 1"));
Console.WriteLine(originator.GetState());
originator.SetState(new State(2, "state 2"));
Console.WriteLine(originator.GetState());
originator.SetState(new State(3, "state 3"));
Console.WriteLine(originator.GetState());
originator.Undo();
originator.Undo();
Console.WriteLine(originator.GetState());
originator.SetState(new State(1, "new state 1"));
Console.WriteLine(originator.GetState());
Console.ReadKey();
}
}
The output from the console application:
Level is 1 with message state 1
Level is 2 with message state 2
Level is 3 with message state 3
Level is 1 with message state 1
Level is 1 with message new state 1
Level is 2 with message state 2
Level is 3 with message state 3
Level is 1 with message state 1
Level is 1 with message new state 1
The pattern seems overly complex when a simple Stack can be used to store state, therefore is a Stack a Memento or could a Stack be a Memento?
Let's take a look and see if I can simplify the pattern:
class OriginatorWithStack {
Stack<State> mementoStack = new Stack<State>();
public void SetState(State state) {
mementoStack.Push(state);
}
public State GetState() {
return mementoStack.Peek();
}
public void Undo() {
mementoStack.Pop();
}
}
Stack<State> mementoStack = new Stack<State>();
public void SetState(State state) {
mementoStack.Push(state);
}
public State GetState() {
return mementoStack.Peek();
}
public void Undo() {
mementoStack.Pop();
}
}
Well the answer is yes I simplified the pattern quite easily by just utilising a Stack data structure, but there's a bit of a problem.
Both my implementations of the originator use the new keyword which leaves the design inflexible to change. Really we need an interface for the memento and this should be injected into the Originator via the Caretaker.
Summary
The memento pattern is a simple pattern but seems a little overly complex when a simple data structure such a stack or linked list could
be used.
This suggests that the pattern really means to use an appropriate data structure to store and encapsulate the state and the memento class should
be abstracted for better flexibility.