Iterator
The iterator pattern
The iterator pattern is a behavioral pattern and a simple pattern to follow. The idea, behind the pattern, is to create an abstraction that makes traversing an aggregate type (list, tree, map,...) the same for all aggregate types.The diagram below shows the pattern, and the Iterator abstraction is exposed to the client, this in-turn, hides the Aggregate from the client.
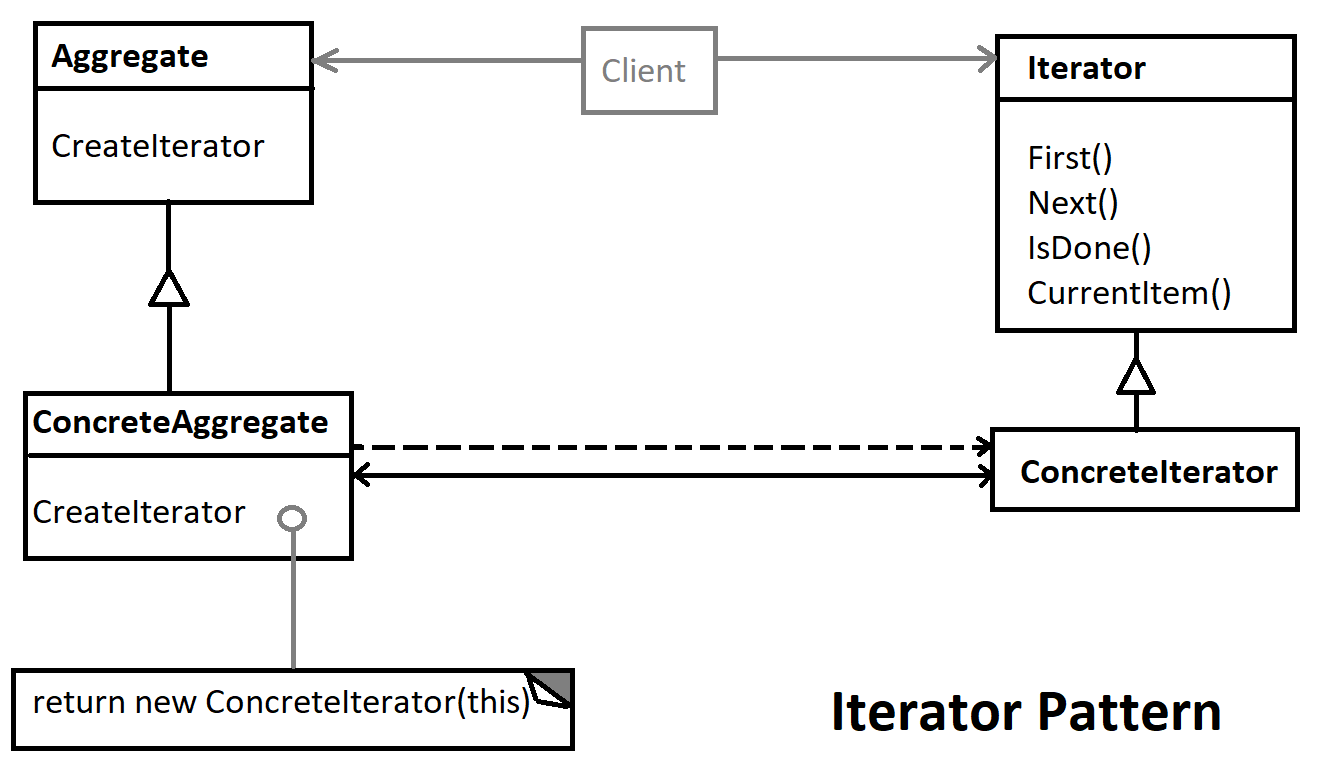
One thing to point out is the factory method the reason for this is to implement a common abstraction, for polymorphism. In that, if I have a number of implementations of list and I'd like the decision on which list to be chosen at runtime, then I can use the factory method CreateIterator() to create the required iterator. This is a little bit out of date and now, we have a rich set of enumerations and storage objects and also we tend to use dependency injection, and the composite root can make the decision on which list should be used.
That said, it's not easy to think of a reason for the iterator pattern so I'll show a simple example, which should show it's worth. Firstly we start with the iterator interface:
interface Iterator- {
void First();
void Next();
bool IsDone();
item CurrentItem();
}
Note that this is defined as an external iterator as the client controls traversal using the
Next(); command (more about internal and external below).
void First();
void Next();
bool IsDone();
item CurrentItem();
}
Here's a simple implementation using the C# List<T> aggregate command:
class ListIterator : Iterator {
private List list;
private int currentIndex;
internal ListIterator(List list) {
this.list = list;
currentIndex = 0;
}
public string CurrentItem() {
return list[currentIndex];
}
public void First() {
currentIndex = 0;
}
public bool IsDone() {
return currentIndex >= list.Count;
}
public void Next() {
currentIndex++;
}
}
And here's another that reverses the list:
private List
private int currentIndex;
internal ListIterator(List
this.list = list;
currentIndex = 0;
}
public string CurrentItem() {
return list[currentIndex];
}
public void First() {
currentIndex = 0;
}
public bool IsDone() {
return currentIndex >= list.Count;
}
public void Next() {
currentIndex++;
}
}
class ReverseIterator : Iterator {
private List list;
private int currentIndex;
internal ReverseIterator(List list) {
this.list = list;
currentIndex = list.Count - 1;
}
public string CurrentItem() {
return list[currentIndex];
}
public void First() {
currentIndex = list.Count - 1;
}
public bool IsDone() {
return currentIndex <= 0;
}
public void Next() {
currentIndex--;
}
}
A program to test the implementations:
private List
private int currentIndex;
internal ReverseIterator(List
this.list = list;
currentIndex = list.Count - 1;
}
public string CurrentItem() {
return list[currentIndex];
}
public void First() {
currentIndex = list.Count - 1;
}
public bool IsDone() {
return currentIndex <= 0;
}
public void Next() {
currentIndex--;
}
}
class Program {
static void Main(string[] args) {
List list = new List {
"apple","banana", "cucumber", "grapefruit", "orange", "pineapple", "tangerine","satsume"
};
ListIterator listIterator = new ListIterator(list);
PrintStrings(listIterator);
ReverseIterator backwardIterator = new ReverseIterator(list);
PrintStrings(backwardIterator);
Console.ReadKey();
}
static void PrintStrings(Iterator iterator) {
while (!iterator.IsDone()) {
Console.Write(iterator.CurrentItem()+" ");
iterator.Next();
}
Console.WriteLine();
}
}
The output is as expected:
static void Main(string[] args) {
List
"apple","banana", "cucumber", "grapefruit", "orange", "pineapple", "tangerine","satsume"
};
ListIterator listIterator = new ListIterator(list);
PrintStrings(listIterator);
ReverseIterator backwardIterator = new ReverseIterator(list);
PrintStrings(backwardIterator);
Console.ReadKey();
}
static void PrintStrings(Iterator
while (!iterator.IsDone()) {
Console.Write(iterator.CurrentItem()+" ");
iterator.Next();
}
Console.WriteLine();
}
}
apple banana cucumber grapefruit orange pineapple tangerine satsume
satsume tangerine pineapple orange grapefruit cucumber banana
satsume tangerine pineapple orange grapefruit cucumber banana
Clearly we've managed to hide the implementation of the aggregate from ...void PrintStrings(..., which has continued printing whatever the iterator wants it to print (we could have a filtered list etc).
The example above is defined as an external iterator, which as mentioned, is an iterator when the client controls the traversal. There's another type called an internal iterator and this is defined as the iterator controlling the traversal (or state) and processing the item as required.
Here's an example:
class InternalIterator {
private List items;
public InternalIterator(List<string> items) {
this.items = items;
}
public void traverse(int numberToTraverse, Action<string> processItem) {
int index = 0;
while (index<numberToTraverse && index<items.Count){
processItem(items[index++]);
}
}
}
Here's the console code to test:
private List
public InternalIterator(List<string> items) {
this.items = items;
}
public void traverse(int numberToTraverse, Action<string> processItem) {
int index = 0;
while (index<numberToTraverse && index<items.Count){
processItem(items[index++]);
}
}
}
static void Main(string[] args) {
List<string> list = new List {
"apple","banana", "cucumber", "grapefruit", "orange", "pineapple", "tangerine","satsume"
};
InternalIterator internalIterator = new InternalIterator(list);
internalIterator.traverse(5, item => Console.Write(item+" "));
Console.ReadKey();
}
And the output:
List<string> list = new List
"apple","banana", "cucumber", "grapefruit", "orange", "pineapple", "tangerine","satsume"
};
InternalIterator internalIterator = new InternalIterator(list);
internalIterator.traverse(5, item => Console.Write(item+" "));
Console.ReadKey();
}
apple banana cucumber grapefruit orange
Summary
The iterator pattern is a simple pattern and hides an aggregate class with a common interface,
thereby the client needs not know which aggregate was used and can process any aggregation that
implements the interface.